Binary Data Handling in JavaScript
Javascript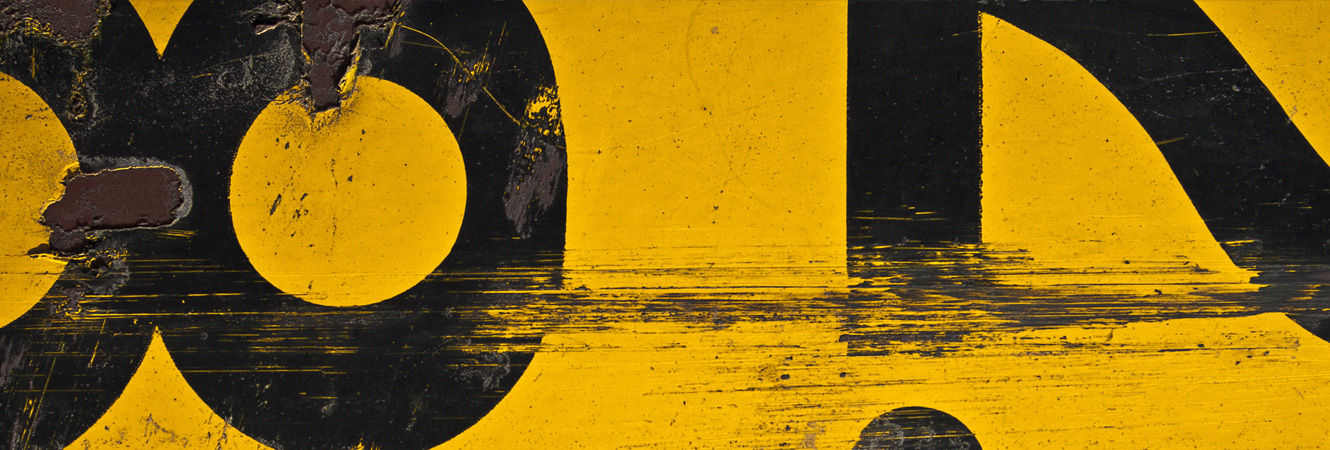
This section provides a concise overview of binary data handling in JavaScript, intended for basic understanding and practical use in conjunction with other APIs. For in-depth applications, further research and detailed implementation are recommended. For additional information, please refer to these resources: link1, link2.
Binary Data: Bits and Bytes
A bit is the smallest unit of information that can be stored or manipulated on a computer. It can represent either 0 or 1. These states are often referred to as true/false, off/on, or yes/no. A byte is a collection of 8 bits.
Computers and network systems operate at the bit level (0s and 1s). This applies to processors, RAM, data storage, network transmission, and more.
While humans typically use the decimal system (base 10), computers use the binary system (base 2). To represent values, computers use combinations of 0s and 1s (e.g., 010010).
Since bits are very small, they are usually grouped into bytes. With 8 bits per byte, you can represent 256 values (0-255).
0 = 00000000 1 = 00000001 ... 255 = 11111111
ArrayBuffers
JavaScript's traditional arrays can hold elements of any type, grow dynamically, and have no size limit. However, they can be slow and memory-intensive. ArrayBuffers and Typed Arrays were introduced in ECMAScript 6 to efficiently handle binary data.
An ArrayBuffer is a fixed-length collection of 8-bit blocks (bytes) that can only store numbers.
For maximum efficiency and flexibility, JavaScript uses buffers and views. An ArrayBuffer stores binary data but cannot access, manipulate, or return it directly. Typed Arrays and DataViews are used to access binary data in a buffer.
Diagram:

Creating an ArrayBuffer:
var buffer = new ArrayBuffer(100); // 100 bytes
Creating an ArrayBuffer with a Typed Array view:
var buffer = new ArrayBuffer(8);
var view = new Int32Array(buffer);
ArrayBuffer Properties and Methods
ArrayBuffer.prototype.byteLength
: Returns the size of the array in bytes.ArrayBuffer.isView(arg)
: Checks if an argument is an ArrayBuffer view.ArrayBuffer.prototype.slice(start, end)
: Creates a new ArrayBuffer from a slice of the original.
Typed Arrays
Typed Arrays are array-like objects that provide a mechanism to access raw binary data. They should not be confused with regular JavaScript arrays.
Typed Arrays are essential for handling binary data in modern web applications, especially with APIs like WebGL, WebSockets, and Web Audio.
Supported APIs:
- File API
- XMLHttpRequest
- Fetch API
- Canvas
- WebSockets
- WebGL
- Web Audio API
Types of Typed Arrays:
- Integer:
Int8Array
,Uint8Array
,Uint8ClampedArray
, etc. - Float:
Float32Array
,Float64Array
Example Typed Array creation:
var f64a = new Float64Array(8);
f64a[0] = 10;
f64a[1] = 20;
f64a[2] = f64a[0] + f64a[1];
DataView
DataView is a low-level interface for reading and writing arbitrary data to a buffer. It allows control over byte order (endianness).
Example DataView usage:
var buffer = new ArrayBuffer(16);
var dv = new DataView(buffer, 0);
dv.setInt16(1, 42);
dv.getInt16(1); // 42
Blob Object
The Blob (Binary Large Object) interface represents raw data, which can be read as an ArrayBuffer. It is used to store binary data and is utilized by other APIs like URL.createObjectURL()
and createImageBitmap()
.
Blob objects allow you to read file size, file type, and slice files into smaller parts.
Creating a Blob:
var aFileParts = ['<a id="a"><b id="b">hey!</b></a>'];
var oMyBlob = new Blob(aFileParts, {type : 'text/html'});
Blob URLs (blob://
) are used to reference Blob objects in memory. They are useful for bypassing server requests and improving performance.
Example Blob URL usage:
var blob = new Blob([arrayBufferWithPNG], {type: "image/png"});
var url = URL.createObjectURL(blob);
var img = new Image();
img.onload = function() {
URL.revokeObjectURL(this.src);
document.body.appendChild(this);
};
img.src = url;
Author of the text: bbosko
Add Your Comment