Resize Observer API
API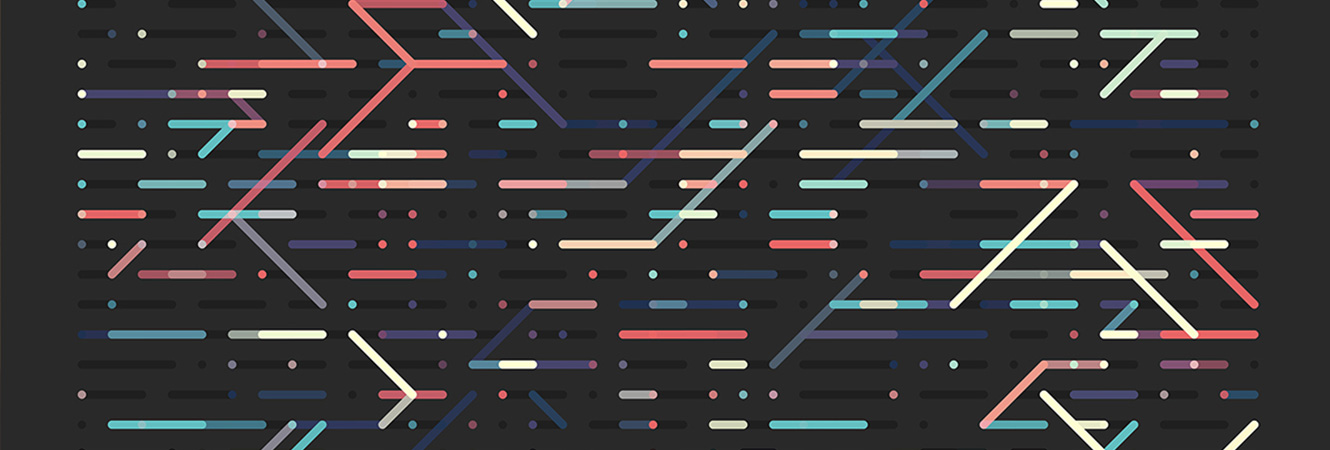
In modern web development, responsive design is paramount. The need to monitor element size changes arises frequently, and that's where the Resize Observer API shines. This API enables efficient tracking of size changes in HTML or SVG elements without incurring performance overhead.
What is the Resize Observer?
The Resize Observer is a JavaScript API that allows you to monitor dimension changes of individual HTML or SVG elements. When an element's size changes, a defined callback function is invoked. This API is highly optimized, meaning it doesn't consume excessive resources, making it ideal for responsive design.
Key Differences from Other Methods
- Resize Event:
- The
window.onresize
event fires repeatedly during window resizing, potentially leading to performance bottlenecks. - Techniques like
debounce
orthrottle
are often necessary to limit the number of callback function invocations. resizeDebounce
executes less often thanResize Observer
.- Debounce example:
let resizeDebounceTime; window.onresize = function () { clearTimeout(resizeDebounceTime); resizeDebounceTime = setTimeout(resizeInit, 200); }; resizeInit();
- The
- matchMedia API:
- This API is better suited for tracking overall window size changes, as it allows the use of media query conditions.
- The callback function is invoked only once when the condition is met.
- Works from IE10.
- Resize Observer:
- Best for tracking size changes of individual elements.
- Doesn't fire as frequently as the Resize Event.
- Fires before the Paint and after the Layout process in the browser, making the callback function an ideal place to make layout adjustments.
- If you change the dimensions of the observed element within the callback function, it won't trigger an infinite loop. The Resize Observer has a mechanism to prevent this. Changes are processed in the next frame.
Advantages of the Resize Observer API
- Efficiency: Optimized for performance, minimizing browser load.
- Precision: Enables tracking of individual element size changes.
- Flexibility: Can be used with various elements (HTML, SVG).
- Fires before the Paint and after the Layout process in the browser.
Disadvantages
- Browser Support: Not supported in Internet Explorer and some mobile browsers (Firefox for Android, Opera Mobile).
- A Polyfill is available to address this issue.
How to Use the Resize Observer
- Create a Resize Observer instance:
const resizeObserver = new ResizeObserver(entries => { // Callback function for (let entry of entries) { console.log(entry); } });
- Observe an element:
resizeObserver.observe(document.getElementById('myElement'));
- Entry Object:
- The callback function receives an array of Entry objects, which contain information about the size changes.
- Key Entry object properties:
entry.borderBoxSize
: Element size including border and padding.entry.contentBoxSize
: Element size excluding border and padding.entry.contentRect
: Object with position and size information.entry.target
: Reference to the observed element.entry.devicePixelContentBoxSize
: The elements content box size, in device pixels.
- Methods:
.observe(el)
: Starts observing an element..unobserve(el)
: Stops observing an element..disconnect()
: Stops observing all elements.
Example Usage
<div id="myElement" style="width: 200px; height: 100px; background-color: lightblue;"></div>
<script>
const resizeObserver = new ResizeObserver(entries => {
for (let entry of entries) {
console.log('Element resized:', entry.contentRect);
}
});
resizeObserver.observe(document.getElementById('myElement'));
</script>
Conclusion
The Resize Observer API is a powerful tool for monitoring element size changes in modern web development. While it has limitations in older browser support, using a polyfill can significantly improve the responsiveness and performance of your web applications.
Author of the text: bbosko
Add Your Comment