JavaScript Form Validacija
Javascript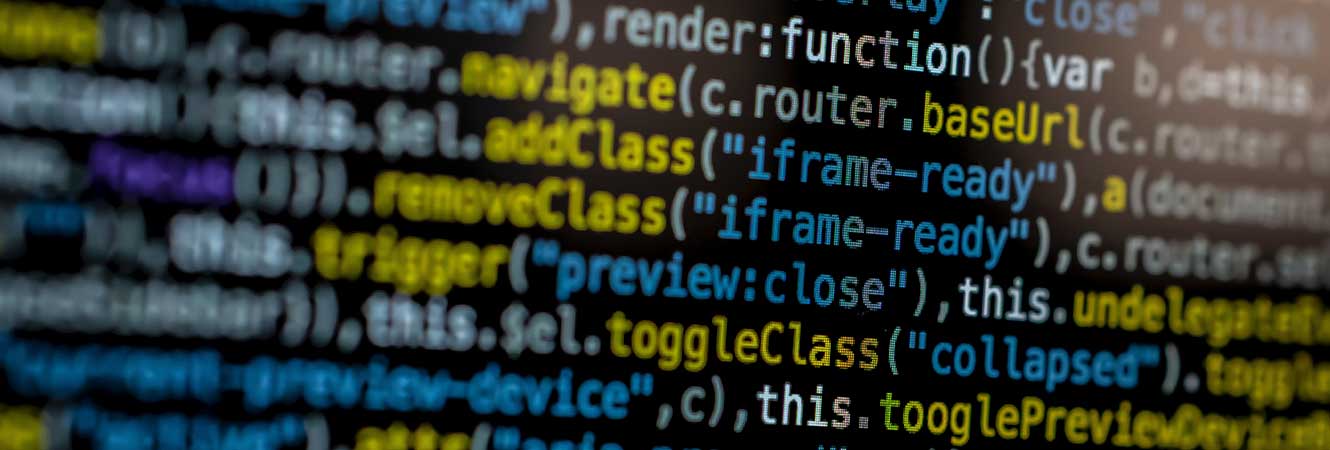
JavaScript Form Validation
When performing JavaScript validation, you first need to disable the default constraint validation performed by the browser by adding the boolean attribute novalidate
to the <form>
element. This prevents the browser from automatically validating the form when the submit button is clicked, disables the display of special styles for valid and invalid forms, and ignores validation-related attributes in form elements.
// You can also dynamically add this attribute using JavaScript and the noValidate property: document.getElementById("myForm").noValidate = true;
In addition to novalidate
, you can use the formnovalidate
attribute in a button or input element with type="submit"
or type="image"
. This allows the form data to be submitted without validation, which is useful for buttons like "Save" that save the current input without submitting the form.
TIP: The novalidate
and formnovalidate
attributes disable the browser's automatic constraint validation but do not prevent the use of the constraint validation API or CSS pseudo-classes such as :valid
and :invalid
. You can still use the API's properties, methods, and pseudo-classes.
If you want to perform advanced validation instead of relying on the limited but easy-to-use HTML validation built into the browser, you need to use JavaScript. The advantage of JavaScript validation is that you can customize the appearance of error messages to ensure consistency across all browsers. Reasons developers prefer JavaScript validation over built-in HTML5 validation include:
- Not all browsers support all HTML5 input types and CSS selectors.
- Error message bubbles use generic text (e.g., “please fill out this field”) and are difficult to style.
:invalid
and:required
styles are applied immediately after the page loads, rather than (optimally) when the user starts interacting with the form.- HTML5 validation has limited capabilities.
Implementing JavaScript Validation
JavaScript validation is typically performed by testing conditions using an if
statement for each form field and each specific condition. These validations are executed in an event handler for the submit
event on the <form>
element. The validation checks can include whether a required field is filled, whether the input matches the allowed character limit, and whether it contains valid characters, among others. If certain fields are invalid, the form submission should be stopped, and the user should be visually informed about the errors and how to correct them. If all the data is valid, the form can be submitted to the server. It’s likely necessary to use the novalidate
attribute on the <form>
element to disable the browser’s default constraint validation.
myForm.onsubmit = checkForm; function checkForm(form) { if (!condition1) { writeError(el, "Error: error message"); form.fieldname.focus(); return false; } if (!condition2) { writeError(el, "Error: error message"); form.fieldname.focus(); return false; } ... return true; }
This function returns true
or false
. If the validation fails, the function returns false
, and the data will not be submitted (returning false
has the same effect as event.preventDefault()
, but only for traditional event creation: el.onevent = function...
. When using addEventListener()
, return false
does not stop the default behavior but only interrupts the execution of the function).
Returning false
also has another use: if a condition is not met, it immediately stops further execution of the function. Only if all conditions are met will the function return true
.
If a condition in the function is not met, the user needs to be informed. Although the alert
command can be used, in practice, it’s better to display the error message on the page near the form field that failed validation.
The focus()
method is used to highlight/select the form element with incorrect values, making it easier for the user to correct them.
Author of the text: bbosko
Add Your Comment