Scope
Javascript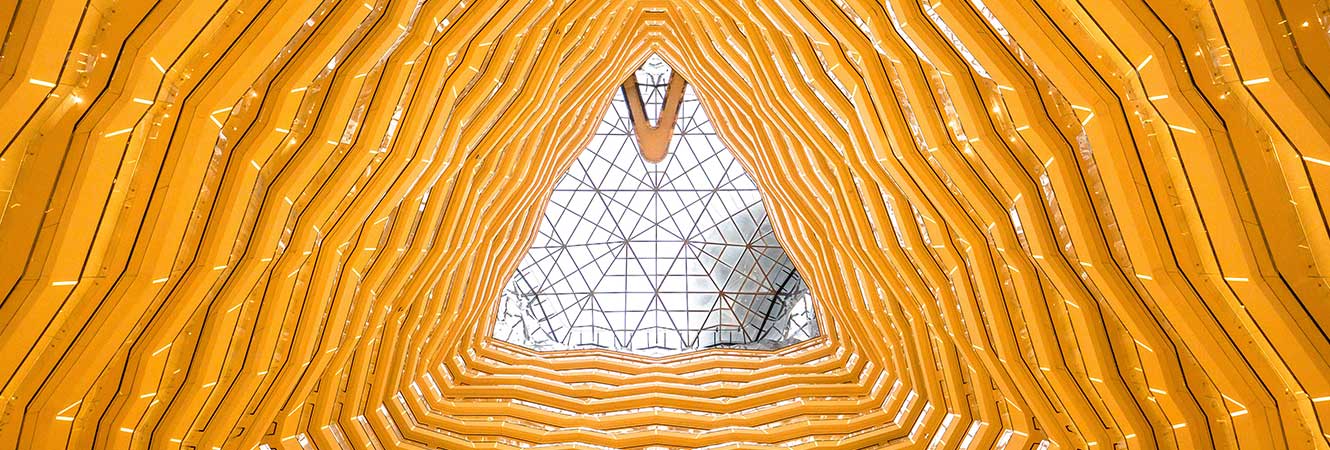
Variables and functions defined outside of a function have a global scope. The global scope in JavaScript is the widest possible scope. It exists across the entire web page, and all variables and functions created in the global scope can be used anywhere on the page.
In addition to variables, functions can also have a global scope. These are functions defined in the code itself, not within another function. Global variables and functions exist throughout the entire page and remain relevant as long as the user does not navigate to another page.
Besides the global scope, there is also the functional or local scope, which limits the use of values to the function in which they are defined. Functions and variables with local scope can access global variables and functions, as well as other local variables and functions defined in the same function. However, they cannot access local variables or functions outside the given function. Similarly, values in the local scope cannot be accessed from the global scope.
var globalVar = "This is a global variable"; function foo() { var localVar = "This is a local variable"; globalVar = localVar; } foo(); alert(localVar); // Throws an error because localVar cannot be accessed outside the function alert(globalVar); // Displays: This is a local variable
After a function finishes execution, all its local variables are destroyed. However, in our example, although localVar
is destroyed, its value is preserved because it was copied to globalVar
before the function completed. Local scope is created by defining functions. Objects, loops, arrays, and similar constructs do not create a local scope, only functions do.
Function parameters (those listed in parentheses) have a local scope and can only be used within the function, not outside of it.
If you have a complex codebase, especially if you have a function inside another function, and that function is inside yet another function, it can be challenging to understand the scope. In such cases, consider creating a diagram with sets to visualize the scopes.
Many programming languages define scope for every block of code, i.e., for any series of statements enclosed in curly braces. However, this is not the case in JavaScript. Regardless of the statement type you use, whether it’s if...else
, a loop, or something similar, all values defined within these statements have a global scope.
var x = 1; console.log(x); // 1 if (true) { var x = 2; console.log(x); // 2 } console.log(x); // 2
How does JavaScript treat and interpret global and local scope?
var globalVar = "Hello from the global variable"; function foo() { alert(globalVar); } foo();
When executing a statement or a function (in this case, foo
), the JavaScript environment searches for the variable globalVar
to execute the function. JavaScript first looks for the variable globalVar
in the local scope, in this case, within the function foo
. Since it cannot find the definition of the variable in the local scope, JavaScript proceeds to the next higher-level scope and continues this process until it reaches the global scope. In our example, the function does not contain other functions and has only one local scope. After searching the local scope, it immediately moves to the global scope, finds the variable identifier, and returns the value.
Author of the text: isladjan
Add Your Comment