Fuction expression
Javascript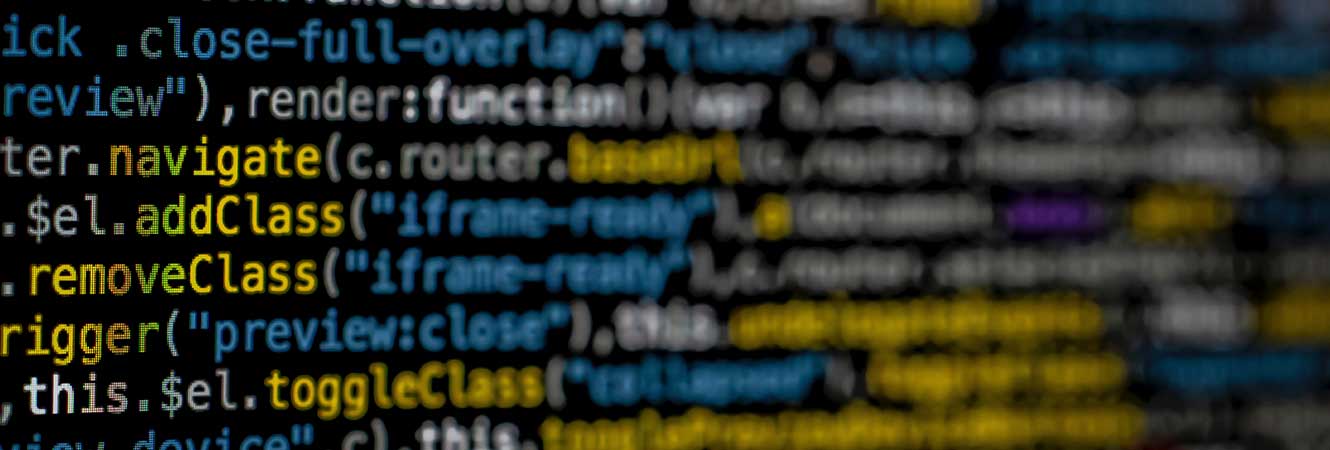
In JavaScript, a function is treated as a value, meaning it can be used in place of any other value.
A function can also be declared by assigning it to a variable, thereby giving the function the name of the variable it is assigned to. In other words, the variable becomes the function. These types of functions are called anonymous functions. However, we can also assign a name to such functions, which is useful when creating self-invoking functions. For these functions, a semicolon is required at the end. This method is used when the function is needed only once or when it needs to execute immediately without being explicitly called (see the example at the end).
var test = function(parameter1) { var example = parameter1; // … return example; }; test(10);
Another way to assign a function to a variable is during a function call by omitting the parentheses. If you omit the parentheses after the function name during the function call, the function itself is treated as a value.
function myFunction(x, y) { return x + y; } var test = myFunction; // the function is assigned without parentheses // In this way, the myFunction function is assigned to the variable test. // The variable test is now a function, and you can use it the same way as myFunction: test(1, 2); // the result is 3 myFunction(1, 2); // the result is 3
When a function is assigned to a variable and called, the call must be placed below the function definition for it to execute. You should be careful when creating multiple interrelated functions to ensure that the call to a function assigned to a variable appears after the function itself. The rule that a function call does not need to be placed below the function applies only to standard functions and their calls.
Author of the text: isladjan
Add Your Comment